Your Ultimate Guide to Understanding SQL (Structured Query Language) The Basic You Need to Know Part II.
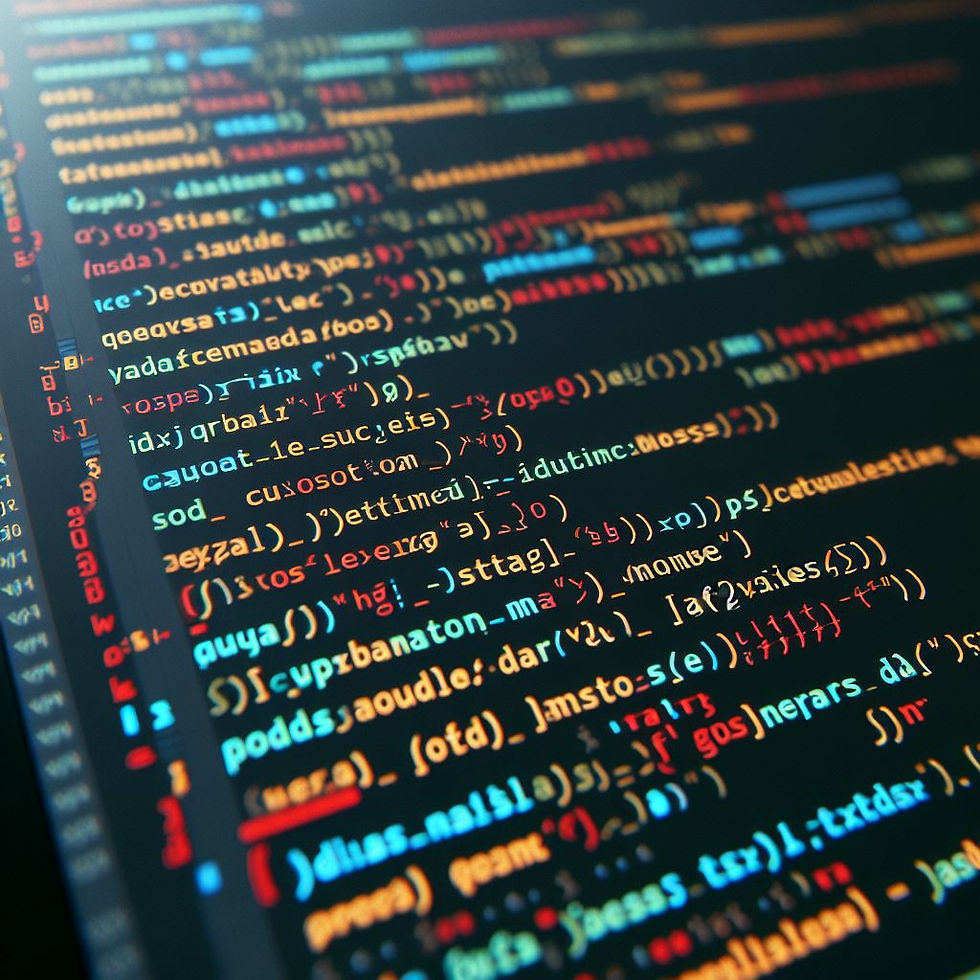
Today, we are going to continue to the other five parts to complete this topic, which includes the following:
Please click this link below to skip to the topic of your interest.
6. Creating, Modifying, and Deleting Tables
6.1 Why do we have to create tables?
Because creating tables is an essential part of database design, tables store data in a structured and organized way. To efficiently and accurately store and retrieve data, it is vital to create tables with correct columns and data types. Creating tables also allows us to establish relationships between different data sets, making extracting meaningful insights from the data more accessible.
How to Create Tables in Microsoft SQL:
1. To begin, please open the Microsoft SQL Server Management Studio.
2. Create a new query and enter the following command to create a new table: CREATE TABLE table_name (column1 data_type, column2 data_type, ...);
3. Enter the columns and data types for the table.
4. Execute the query to create the table.
Example queries of creating a new table in SQL:
CREATE TABLE Customers (
CustomerID int NOT NULL PRIMARY KEY,
CustomerName varchar(255) NOT NULL,
Address varchar(255) NOT NULL,
City varchar(255) NOT NULL,
State varchar(255) NOT NULL,
ZipCode varchar(255) NOT NULL
);
Result = The query result is a new customer table with the specified columns and data types.
6.2 Why do we have to modify the table?
Modifying tables is an essential part of database design. As data requirements change, tables may need to be modified to accommodate the new requirements. Modifying tables can improve query performance and make the data more accessible. Modifying tables can also ensure data consistency and accuracy.
How to Modifying Tables in Microsoft SQL:
1. To open the Microsoft SQL Server Management Studio, please follow these steps:
1.1 Go to the Windows Start menu.
1.2 Search for "Microsoft SQL Server Management Studio".
1.3 Click on the app to open it.
2. Create a new query and enter the following command to modify a table: ALTER TABLE table_name ADD/MODIFY/DROP column_name data_type;
3. Enter the column and data type for the modification.
4. Execute the query to modify the table.
Example quires of modifying a table in SQL:
ALTER TABLE Customers
ADD EmailAddress varchar(255) NOT NULL;
Result = The query result is an updated table called Customers with an additional column called EmailAddress with the specified data type.
6.3 When do we have to delete the table?
Deleting tables is an integral part of database design. Tables should be deleted when they are no longer needed or when the data is no longer valid. Deleting tables can free up storage space and improve query performance.
How to Deleting Tables in Microsoft SQL:
1. To begin, please open Microsoft SQL Server Management Studio.
2. Create a new query and enter the following command to delete a table: DROP TABLE table_name;
3. Execute the query to delete the table.
Example quires of deleting a table in SQL:
DROP TABLE Customers;
Result = The result of the query is a deleted table called Customers.
***cautions for deleting table***
Some critical cautions are required when deleting a table. Deleting a table will delete all the associated data, so it is crucial to ensure the data is no longer needed before deleting it. Providing other tables or queries that do not reference the table is essential, as this can cause errors or unexpected results.
7. Inserting, Updating, and Deleting Data
7.1 What is Inserting, Updating, and Deleting Data?
Inserting data is the process of adding new data to a database. This process can be done using the INSERT statement in SQL. The INSERT statement is used to add one or more records to a table. It is INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...).
Updating data is the process of modifying existing data in a database. This activity can be done using the UPDATE statement in SQL. The UPDATE statement is an SQL command that allows you to modify one or more records in a table by changing the values of specific columns. It is commonly used to update existing data in a table. It takes the form of UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition.
Deleting data is the process of removing data from a database. This function can be done using the DELETE statement in SQL. The DELETE statement deletes one or more records from a table. It takes the form of DELETE FROM table_name WHERE condition.
7.2 Why do we have to Inserting, Updating, and Deleting Data?
Inserting data is necessary when adding new records to a database. This function can add customers, products, and orders to the database. Inserting data is also helpful for keeping track of changes over time, such as customer orders or inventory levels.
Updating data is necessary when modifying existing records in a database. This update can modify customer information, product prices, and order details. Updating data is also helpful for keeping track of changes over time, such as customer orders or inventory levels.
Deleting data is necessary when removing records from a database. This activity can delete outdated or inaccurate documents, such as old customer orders or expired products. Deleting data is also helpful for keeping the database organized and avoiding data duplication.
***To clarify between delete data vs. delete table***
Deleting data is the process of removing specific records from a table in a database. This command is done using the DELETE statement in SQL. Deleting a table removes an entire table and all its associated data from a database. This activity is done using the DROP statement in SQL.
7.3 The example of queries of inserting, Updating, and Deleting Data
Example of an INSERT statement in SQL:
INSERT INTO Customers (CustomerName, Address, City, State, ZipCode)
VALUES ('John Doe', '123 Main Street', 'New York', 'NY', '12345');
This statement will add a new record to the Customers table with the specified values.
Example of an UPDATE statement in SQL:
UPDATE Customers
SET Address = '456 Main Street'
WHERE CustomerID = 1;
This statement will modify the record with the CustomerID of 1 in the Customers table and set the Address to '456 Main Street'.
Example of a DELETE statement in SQL:
DELETE FROM Customers
WHERE CustomerID = 1;
This statement will delete the record with the CustomerID of 1 from the Customers table.
8. Working with Views, Stored Procedures, and Triggers
8.1 Why do we have to work with Views, Stored Procedures, and Triggers in SQL?
Using views, stored procedures, and triggers can enhance query performance and improve data accessibility. Views create virtual tables based on existing tables, simplifying complex queries. Stored procedures store frequently used queries, which can be executed with a single command. Triggers automatically manage a query or set of queries when certain conditions are met. To improve the efficiency and maintainability of their code, developers can utilize views, stored procedures, and triggers. These tools help streamline the development process and make it easier to manage complex codebases.
8.2 Example of queries of Views, Stored Procedures, and Triggers
Example of a view in SQL:
CREATE VIEW CustomersByCity
AS
SELECT CustomerName, City
FROM Customers;
Result = This view will create a virtual table called CustomersByCity which will return the CustomerName and City columns from the Customers table.
Example of a stored procedure in SQL:
CREATE PROCEDURE GetCustomersByCity
(@City VARCHAR(255))
AS
BEGIN
SELECT CustomerName
FROM Customers
WHERE City = @City
END;
Result = This stored procedure will create a procedure called GetCustomersByCity which will return the CustomerName column from the Customers table where the City matches the specified parameter.
Example of a trigger in SQL:
CREATE TRIGGER UpdateCustomerName
ON Customers
AFTER UPDATE
AS
BEGIN
UPDATE Orders
SET CustomerName = (SELECT CustomerName FROM Customers WHERE CustomerID = Orders.CustomerID)
END;
Result = This trigger will create an UpdateCustomerName, automatically updating the CustomerName column in the Orders table whenever the Customers table is updated.
Mastering views, stored procedures, and triggers is considered an advanced skill in SQL. These techniques help improve query performance and make data more accessible. Before attempting to work with these techniques, it is vital to have a good understanding of SQL and database design.
9. Indexing Data and Optimizing Queries
9.1 What are Indexing Data and Optimizing Queries?
Indexing data and optimizing queries is the process of making changes to the database structure and the queries used to access the data to improve query performance. Indexing data involves adding indexes to tables to improve the performance of queries. Optimizing queries applies to changing the queries to reduce the database's work.
Moreover, indexing data and optimizing queries can be advanced. It involves understanding database structure, query performance, and query optimization techniques. It also requires a thorough understanding of the data and the queries used.
9.2 Example of the indexing
An example of indexing data is creating an index on a table to speed up searches on a column. For example, if you have a table of orders and want to search for orders based on the customer ID, you could create an index on the customer ID column to speed up the query. The index will tell the database engine which rows to look at first, reducing the database's work.
9.3 How to optimizing queries
An example of optimizing queries is using the most efficient query possible for the task. For example, when searching for information, a SELECT statement with a WHERE clause is usually more efficient than a SELECT * statement. Correct data types, indexes, and JOINs can also help optimize queries. Finally, using prepared statements can also help reduce the number of queries that need to be run.
I will give you some examples of optimizing queries:
1. Using a SELECT statement with a WHERE clause instead of a SELECT * statement.
2. Use the correct data types (e.g., INT instead of VARCHAR).
3. Creating indexes on columns to improve query performance.
4. Using JOINs instead of subqueries when possible.
5. Using prepared statements to reduce the number of queries.
6. Optimizing GROUP BY and ORDER BY clauses.
7. Avoid unnecessary columns and joins in queries.
10. Best Practices for Writing Efficient SQL Queries
10.1 What is the best practice for writing efficient SQL Queries?
Best practices for writing efficient SQL queries include using the correct data type for each column, using appropriate indexing for queries, using wildcards and LIKE clauses to reduce the size of the result set, using UNION ALL instead of UNION when combining queries, using joins instead of subqueries when possible, avoiding using ORDER BY on large result sets, avoiding using cursors and temporary tables, using EXISTS and NOT EXISTS instead of IN and NOT IN, using prepared statements to reduce the number of queries, and using proper whitespace and indentation to make queries more straightforward to read.
I will break down into 10 best practices for writing efficient SQL Queries:
1. Use the correct data type for each column.
2. Use appropriate indexing for queries.
3. Use wildcards and LIKE clauses to reduce the size of the result set.
4. Use UNION ALL instead of UNION when combining queries.
5. Use joins instead of subqueries when possible.
6. Avoid using ORDER BY on large result sets.
7. Avoid using cursors and temporary tables.
8. Use EXISTS and NOT EXISTS instead of IN and NOT IN.
9. Use prepared statements to reduce the number of queries.
10. Use proper whitespace and indentation to make queries more straightforward to read.
Please let me know if you need more explanation of best practices for writing efficient SQL. I will explain in a separate topic because it requires more details added. For example, when writing efficient SQL queries, best practices include
using the correct data type,
indexing queries,
using wildcards and LIKE clauses and UNION ALL,
using joins instead of subqueries,
avoiding ORDER BY on large result sets,
avoiding cursors and temporary tables,
using EXISTS and NOT EXISTS instead of IN and NOT IN,
using prepared statements and
using proper whitespace and indentation.
Conclusion
Data analysts and SQL are closely related and often used together. SQL is a powerful language for managing, storing, and retrieving database data. Data analysts use SQL to query and manipulate large amounts of data, enabling them to find meaningful insights and trends. SQL is a vital tool for data analysts and is often utilized with other analytical tools to make sense of data.
Data analysts use SQL to explore and analyze data by creating queries that allow them to filter, sort, and join tables. They use SQL to create reports and visualizations for informed decisions. SQL is an essential skill for data analysts, and they must be familiar with the language and its capabilities to succeed in their work.
***These examples apply to all type of SQL, including Microsoft SQL***
Comments